Something like this? (only underwater)
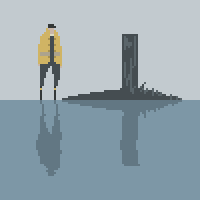
I was working on the exact same thing. I did this in HaxePunk but it should work just as well with Flash, but doing this a lot can be quite intensive and maybe slow down your game a bit. Use it with caution.
Here’s how you do it:
You basicly have to get the BitmapData of your entities/graphics, then do some manipulation to it with BitmapData.copyPixels();
For example you could offset all pixels based on a sine curve (which would get you a nice ripple effect, i use something more random where I calculate an Array of offsets with different heights and then animate that downwards to make it seem moving). And then render this BitmapData.
I don’t have time to check it but something along these lines:
public function MyEntity()
{
//Get the BitmapData of your sprite
var mybitmapdata:BitmapData = FP.getBitmap(IMAGE_TO_APPLY_RIPPLE_EFFECT_ON);
graphic = new Image(mybitmapdata);
}
override public function update():void
{
//You can also do this in the render() function
for (var i:int = 0; i < mybitmapdata.height; i++) //Go through each row of pixels in mybitmapdata
{
//and give it a random offset (you could also use sine curve, or other)
mybitmapdata.copyPixels(FP.getBitmap(IMAGE_TO_APPLY_RIPPLE_EFFECT_ON), new Rectangle(0, i, mybitmapdata.width, 1), new Point(FP.choose(-1,0,1), i));
//so we copy a rectange (line) from the original image and offset it by either -1, 0, or 1 pixel
}
super.update();
}
You can also do this to the whole screen buffer, FP.buffer, or group multiple images together in one BitmapData so you don’t have to do it individually to each entity/graphic.